本文基本都是 ctrl + cv,用于学习记录,由于历史遗留问题发至博客记录。
Salsa20
Salsa20是一种流加密算法,它使用一个称为Salsa20核心的函数来加密或解密数据。下面是Salsa20算法的详细解释,包括加密和解密流程。
- Salsa20算法的核心函数
Salsa20核心函数是一个置换函数,它将输入的128位密钥和随机的64位初始向量(IV)作为输入,然后生成一个256位的密钥流。密钥流是通过对128位输入块进行加密和异或操作生成的。下面是Salsa20核心函数的数学表达式:
Salsa20核心函数输入:密钥K(128位)、初始向量IV(64位)、计数器C(64位)。
Salsa20核心函数输出:密钥流(256位)。
Salsa20核心函数的伪代码:
1 | Salsa20(K, IV, C) |
其中,constants是一个常量数组,QuarterRound是一个四元运算函数,它对输入的四个32位数进行加密和异或操作,生成四个32位数作为输出。
- Salsa20算法的加密流程
Salsa20算法使用密钥流对明文进行加密。加密流程可以分为以下步骤:
(1)将明文分成若干个128位的块。
(2)对每个块进行密钥流加密,使用异或操作将密钥流和明文块进行混合。
(3)将加密后的密文块组合在一起,形成加密后的数据。
下面是Salsa20算法加密流程的数学表达式:
Salsa20加密流程输入:明文M(n * 128位)、密钥K(128位)、初始向量IV(64位)。
Salsa20加密流程输出:密文C(n * 128位)。
Salsa20加密流程的伪代码:
1 | Salsa20Encrypt(M, K, IV) |
其中,n表示明文块的数量,M[i]表示第i个明文块,C[i]表示第i个密文块。
- Salsa20算法的解密流程
Salsa20算法使用密钥流对密文进行解密。解密流程可以分为以下步骤:
(1)将密文分成若干个128位的块。
(2)对每个块进行密钥流解密,使用异或操作将密钥流和密文块进行混合。
(3)将解密后的明文块组合在一起,形成解密后的数据。
下面是Salsa20算法解密流程的数学表达式:
Salsa20解密流程输入:密文C(n * 128位)、密钥K(128位)、初始向量IV(64位)。
Salsa20解密流程输出:明文M(n * 128位)。
Salsa20解密流程的伪代码:
1 | Salsa20Decrypt(C, K, IV) |
其中,n表示密文块的数量,C[i]表示第i个密文块,M[i]表示第i个明文块。
综上所述,Salsa20算法是一种高效、安全的流加密算法,它可以用于数据通信、数据存储等多种场景。在实际应用中,需要注意密钥的生成、密钥流的保密性等问题,以确保数据的安全性。
1 |
|
但感觉这代码怪怪的,和文章看到的不太一样,没找到个喜欢的代码
1 | from Crypto.Cipher import Salsa20 |
ChaCha20
一、简介
Chacha20流密码经常和Poly1305消息认证码结合使用,被称为ChaCha20-Poly1305,由Google公司率先在Andriod移动平台中的Chrome中代替RC4使用,由于其算法精简、安全性强、兼容性强等特点,目前Google致力于全面将其在移动端推广
二、初始化矩阵
ChaCha20加密的初始状态包括了包括了
1、一个128位常量(Constant)
常量的内容为0x61707865,0x3320646e,0x79622d32,0x6b206574.)
2、一个256位密钥(Key)
3、一个64位计数(Counter)
4、一个64位随机数(Nonce)
一共64字节其排列成4 * 4的32位字矩阵如下所示:(实际运算为小端)

ChaCha20.h
1 |
|
ChaCha.c
1 |
|
main.c
1 |
|
测试结果
1 | Encrypt: 0x9A, 0x66, 0x7F, 0x13, 0xB8, 0xF2, 0x4A, 0xA2, 0x74, 0x8C, 0x1C, 0x63, 0xAC, 0xF0, 0x4A, 0x32, 0xD0, 0x48, 0x50, 0x50, 0x84, 0x54, 0x4, 0xC, 0xD0, 0x48, 0x50, 0x50, 0x84, 0x54, 0x4, 0xC, |
python版本
1 | import json |
开摆,等哪题碰到这种题再测
Reference
https://www.cnblogs.com/lordtianqiyi/articles/16822639.html
https://openprompt.co/conversations/1861
https://pycryptodome.readthedocs.io/en/latest/src/cipher/salsa20.html
https://pycryptodome.readthedocs.io/en/latest/src/cipher/chacha20.html
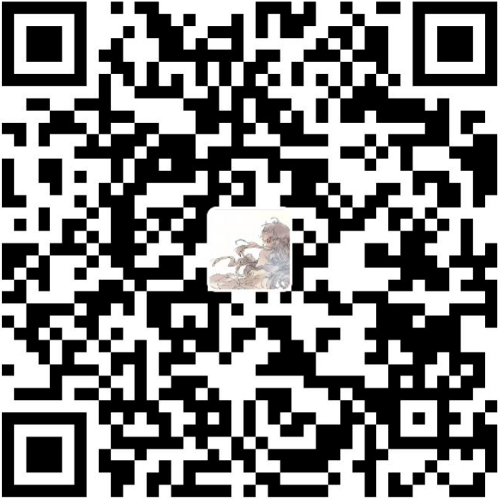
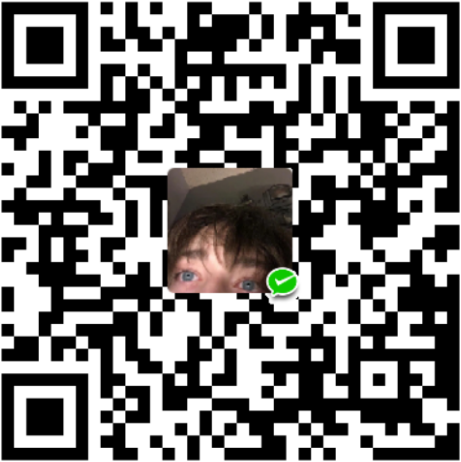
About this Post
This post is written by P.Z, licensed under CC BY-NC 4.0.